Given a reference of a node in a connected undirected graph, return a deep copy (clone) of the graph. Each node in the graph contains a val (
int
) and a list (List[Node]
) of its neighbors.
Example:
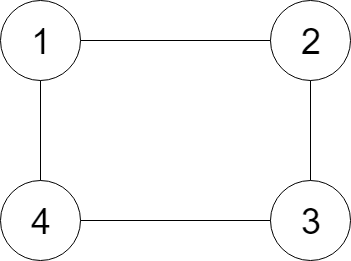
Input: {"$id":"1","neighbors":[{"$id":"2","neighbors":[{"$ref":"1"},{"$id":"3","neighbors":[{"$ref":"2"},{"$id":"4","neighbors":[{"$ref":"3"},{"$ref":"1"}],"val":4}],"val":3}],"val":2},{"$ref":"4"}],"val":1} Explanation: Node 1's value is 1, and it has two neighbors: Node 2 and 4. Node 2's value is 2, and it has two neighbors: Node 1 and 3. Node 3's value is 3, and it has two neighbors: Node 2 and 4. Node 4's value is 4, and it has two neighbors: Node 1 and 3.
Note:
- The number of nodes will be between 1 and 100.
- The undirected graph is a simple graph, which means no repeated edges and no self-loops in the graph.
- Since the graph is undirected, if node p has node q as neighbor, then node q must have node p as neighbor too.
- You must return the copy of the given node as a reference to the cloned graph.
Notes:
The key is to avoid multiple copies of the same node. So we can used a hash map to build up a one-to-one relationship.
Either BFS or the DFS will work.
See the code below:
/* // Definition for a Node. class Node { public: int val; vector<Node*> neighbors; Node() {} Node(int _val, vector<Node*> _neighbors) { val = _val; neighbors = _neighbors; } }; */ class Solution { public: Node* cloneGraph(Node* node) { if(!node) return node; Node* copy = new Node(node->val, {}); unordered_map<Node*, Node*> mp; mp[node] = copy; queue<Node*> q; q.push(node); while(q.size()) { auto t = q.front(); q.pop(); for(auto &a : t->neighbors) { if(!mp.count(a)) { mp[a] = new Node(a->val, {}); q.push(a); } mp[t]->neighbors.push_back(mp[a]); } } return copy; } };
No comments:
Post a Comment